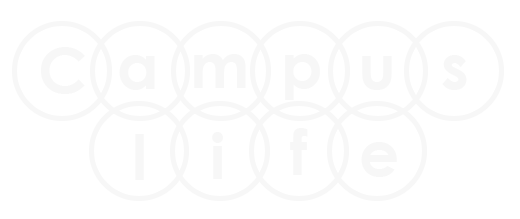
"KEEP LEARNING, DON'T STOP."
"KEEP LEARNING, DON'T STOP."
An Armstrong number of three digits is an integer such that the sum of the cubes of its digits is equal to the number itself.
Formula:- n! = n x (n - 1) x (n - 2) x .... x 1
7 = 7^1 371 = 3^3 + 7^3 + 1^3(27+343+1) 8208 = 8^4 + 2^4 +0^4 + 8^4 (4096 + 16 + 0 + 4096)
#include<iostream.h> #include<conio.h> //ARMSTRONG NUMBERS PROGRAM void main() { int n, a, num, temp, sum=0; clrscr(); cout<<"ENTER A NUMBER:- "; cin>>n; num = n; do { temp = n%10; a = temp*temp*temp; sum = sum + a; n = n/10; } while(n!=0); if(sum == num) { cout<<num<<" IS ARMSTRONG NUMBER."; } else { cout<<num<<" NOT A ARMSTRONG NUMBER."; } getch(); }
ENTER A NUMBER:- 371 371 IS ARMSTRONG NUMBER.