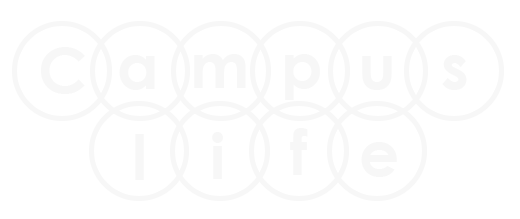
"KEEP LEARNING, DON'T STOP."
"KEEP LEARNING, DON'T STOP."
Array is like a Basket. We carried basket when we are going to market. In a basket we can store apple, banana, vegetables etc. Here array is same like basket.
In array we can store homogeneous (Similar type) type of data. An array is an indexed collection of homogeneous data element (value). Every value is store in array based on indexed. In array we can store multiple values.
4 types of array are in Java language.
In single dimensional array we can store only single data type value. Single variable but one " [ ] " subscript.
We can't initialize the size of array at the time of declaration.
In two ways we can initialize the 1D array
Further in compile time initialization we have two types initialization.
int a[] = {5,4,3}; int []a = {5,4,3}; int a[3] = {5,4,3}; //invalid declaration
int a[] = new int[3]; a[0] = {2}; a[1] = {6}; a[2] = {3};
We initialize the value of array at runtime.
import java.util.*; class Test7 { public static void main(String arg[]) { Scanner sc = new Scanner(System.in); int a[] = new int[5]; int i; for(i=0; i<a.length; i++) { System.out.print("Elements a["+i+"] : "); a[i] = sc.nextInt(); } } }
Enter the Size of Array Elements a[0] : 5 Elements a[1] : 6 Elements a[2] : 5 Elements a[3] : 3 Elements a[4] : 1
In 2D or multi-dimensional array we can store multiple data value with single variable in the form of row and column with two ( [row][column] ) subscript. Array brackets are called subscript.
In java 2D array implemented as an array of array.
In two ways we can initialize the 2D array
Further in compile time initialization we have two types initialization.
int a[][] = {{1,2},{3,4},{5,6}}; int [][]a = {{1,2},{3,4},{5,6}}; int a[3][] = {{1,2},{3,4},{5,6}}; //invalid declaration
int a[][] = new int[3][2]; a[0][0] = 1; a[0][1] = 2; a[1][0] = 3; a[1][1] = 4; a[2][0] = 5; a[2][1] = 6;
We initialize the value of array at runtime.
import java.util.*; class Test8 { public static void main(String arg[]) { System.out.println("Enter the Size of Array"); Scanner sc = new Scanner(System.in); int a[][] = new int[3][3]; int i, j; for(i=0; i<a.length; i++) { for(j=0; j<3; j++) { System.out.print("Enter elements a["+i+"]["+j+"] : "); a[i][j] = sc.nextInt(); } } for(i=0; i<a.length; i++) { for(j=0; j<3; j++) { System.out.print(a[i][j]+" "); } System.out.println(); } } }
Enter elements a[0][0] : 1 Enter elements a[0][1] : 2 Enter elements a[0][2] : 3 Enter elements a[1][0] : 4 Enter elements a[1][1] : 5 Enter elements a[1][2] : 6 Enter elements a[2][0] : 7 Enter elements a[2][1] : 8 Enter elements a[2][2] : 9 1 2 3 4 5 6 7 8 9
In 3D or multi dimensional array we can store multiple data value with single variable in the form of row and column with three ( [ ][ ][ ] ) subscript. Array brackets are called subscript.
Very rare case people use 3D array. Mostly we use 1D or 2D array in Java. In java 3D array implemented as an array of array
In two ways we can initialize the 3D array or multi dimensional
Further in compile time initialization we have two types initialization.
int a[][][] = {{1,2},{5,6},{7,8}};
int a[][][] = new int[3][2][2]; a[0][0][0] : 1 a[0][0][1] : 2 a[0][1][0] : 3 a[0][1][1] : 4 a[1][0][0] : 1 a[1][0][1] : 2 a[1][1][0] : 3 a[1][1][1] : 4 a[2][0][0] : 1 a[2][0][1] : 2 a[2][1][0] : 3 a[2][1][1] : 4
We initialize the value of array at runtime.
import java.util.*; class Test9 { public static void main(String arg[]) { Scanner sc = new Scanner(System.in); int a[][][] = new int[3][2][2]; int i, j, k; for(i=0; i<a.length; i++) { for(j=0; j<2; j++) { for(k=0; k<2; k++) { System.out.print("Enter elements a["+i+"]["+j+"]["+k+"] : "); a[i][j][k] = sc.nextInt(); } } } System.out.println("Displaying Result in Matrix form: "); for(i=0; i<a.length; i++) { for(j=0; j<2; j++) { for(k=0; k<2; k++) { System.out.print(a[i][j][k]+" "); } } System.out.println(); } } }
Displaying Result in Matrix form: 1 2 3 4 5 6 7 8 9 10 11 12
Some time we create an array in java without name such type nameless array called anonymous array.
new data-type[] {elements};
//pending
//pending