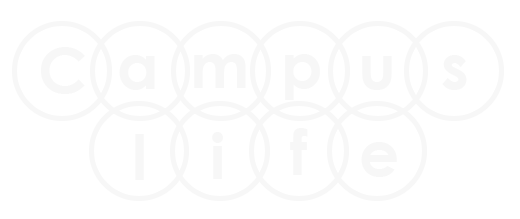
"KEEP LEARNING, DON'T STOP."
"KEEP LEARNING, DON'T STOP."
Constructor is like a method, but it is not exactly a method. It is special type of method which is called by JVM (Java Virtual Machine) automatically at the time object creation. In java class we have default constructor which is created by compiler at the time of compilation.
Constructor is used to initialize the instance variables in a java class.
this keyword is use to differentiate the same instance variable name and constructor parameter name. In below example constructor parameter name and instance variable name are same. To solve the this problem we use this keyword. You can use this keyword with any of the variables.
class A { int age; public A(int age) { //use of this keyword. this.age = age; } }
In java every class have one default constructor which created by the compiler at the time of compilation. By default compiler add one default constructor look like a method. Which is called by JVM (Java Virtual Machine) at the time of compilation.
class A { public void book() { } } class Test1 { public static void main(String arg[]) { System.out.println("Default constructor program.."); A obj = new A(); obj.book(); } }
In above example we didn't created any constructor. But when you will run it with javap tool. It will show you default constructor which is automatically created by JVM at the time of compilation.
1. First compile the above program by using cmd. javac Test1.java
C:\Users\Nature\Desktop\Java>javac Test1.java
After compiling the Test1.java file one Test1.class file created.
2. Now compile the Test1.class file in cmd using javap tool.
C:\Users\Nature\Desktop\Java>javap Test1.class
C:\Users\Nature\Desktop\Java>javap Test1 Compiled from "Test1.java" class Test1 { Test1(); public static void main(java.lang.String[]); }
Now see the above output of Test1.class file. A Test1() default constructor automatically created by compiler at the time of compilation.
A constructor have no parameter is called zero parameterized constructor.
class A { int num; //zero parameterized constructor. public A() { num = 10; System.out.println("Num = "+num); } } class Test2 { public static void main(String arg[]) { A obj = new A(); } }
Num = 10
A constructor have parameter is called parameterized constructor.
class A { int num; //parameterized constructor. public A(int i) { num = i; System.out.println("Num = "+num); } } class Test2 { public static void main(String arg[]) { A obj = new A(20); } }
Num = 20
Constructor overloading is same like a method overloading. A constructor with same name but with different parameters is called Constructor overloading.
class A { int num; String name; public A(int i) { num = i; System.out.println("Num = "+num); } public A(String b) //constructor overloading. { name = b; System.out.println("Name = "+name); } } class Test2 { public static void main(String arg[]) { A obj = new A(10); A obj1 = new A("Campuslife"); } }
Num = 10 Name = Campuslife