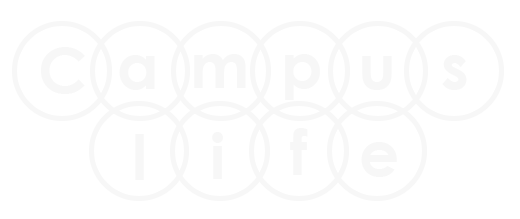
"KEEP LEARNING, DON'T STOP."
"KEEP LEARNING, DON'T STOP."
Naming convention are nothing but rules for writing names in java. Which may be class name, variables name or method name etc. For that Sun Microsystem given some rules.
This rules are recommended but not mandatory.
Every class you have to start with capital letter.
//Naming Convention 1 class Employee { } //Naming Convention 2 class Customer { } //Naming Convention 3 class CourierTracking { }
Every variables we have to start with small word. Single letter start with lower and second word must with capital.
gender, age, panNo, customerName, addressLine, phoneNumber;
Method exactly same as a variable name. Every method we have to start with small word. Single letter start with lower and second word must with capital.
display() add() withdrow() getStatus() getId() personeDetails() areaAddress()
All words should be lower case.
if, else, switch, case, for, etc....
All words should be lower case with ( . ) symbol.
import java.util; import java.io; import java.lang; etc...
Static constant variables represent in upper letter.
thread.MAX_PRIORITY thread.MIN_PRIORITY thread.NORM_PRIORITY
class Student { int studentId= 123, age = 25, phoneNumber = 99157; String studentName = "Manzar"; public void getDetails() { System.out.println("Student Id = "+studentId); System.out.println("Student Name = "+studentName); System.out.println("Student Age = "+age); System.out.println("Student Phone Number = "+phoneNumber); } } class Record { public static void main(String arg[]) { Student std = new Student(); std.getDetails(); } }
Student Id = 123 Student Name = Manzar Student Age = 25 Student Phone Number = 99157