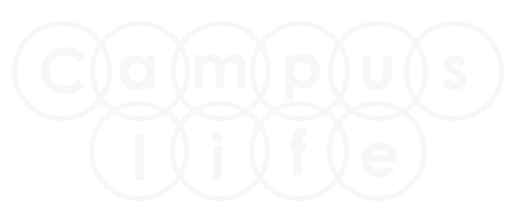
"KEEP LEARNING, DON'T STOP."
"KEEP LEARNING, DON'T STOP."
In this section you will learn how to check whether a number Armstrong or not. In this program we are taking the input from the user.
An Armstrong number of three digits is an integer such that the sum of the cubes of its digits is equal to the number itself.
7 = 7^1 371 = 3^3 + 7^3 + 1^3(27+343+1) 8208 = 8^4 + 2^4 +0^4 + 8^4 (4096 + 16 + 0 + 4096)
Scanner function is use to take the input from user in java.
import java.util.Scanner; class Armstrong { public static void main(String arg[]) { int n, a, num, temp, sum=0; Scanner sc = new Scanner(System.in); System.out.println("ENTER A NUMBER:- "); n = sc.nextInt(); num = n; do { temp = n%10; a = temp*temp*temp; sum = sum + a; n = n/10; } while(n!=0); if(sum == num) { System.out.println(num+" IS ARMSTRONG NUMBER."); } else { System.out.println(num+" NOT A ARMSTRONG NUMBER."); } } }
ENTER A NUMBER:- 371 371 IS ARMSTRONG NUMBER.
Advertisment