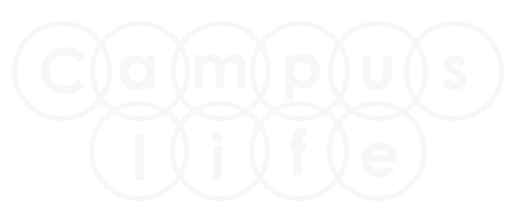
"KEEP LEARNING, DON'T STOP."
"KEEP LEARNING, DON'T STOP."
In this section you will learn how to reverse the numbers in Java without using loop. We will use two variable for reverse the numbers and simple mathematic operation. In this program we are taking the input from user.
Reverse mean shifting the first value to last value and shifting the last value to first value.
Let’s suppose a user will input a number ‘134’, then we will perform reverse operation on it.
Scanner function is use to take the input from user in java.
import java.util.Scanner; class Reverse { public static void main(String arg[]) { int num, temp; Scanner sc = new Scanner(System.in); System.out.println("ENTER THE FIRST NUMBER:-"); num = sc.nextInt(); System.out.println("BEFORE REVERSE VALUE IS:- "+num); System.out.print("AFTER REVERSE RESULT IS:- "); temp = num%10; System.out.print(temp); temp = num/10; temp = temp%10; System.out.print(temp); temp = num/10; temp = temp/10; System.out.print(temp); } }
BEFORE REVERSE VALUE IS:- 134 AFTER REVERSE RESULT IS:- 431
Advertisment