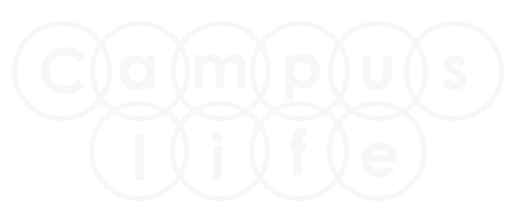
"KEEP LEARNING, DON'T STOP."
"KEEP LEARNING, DON'T STOP."
In this section you will learn how to check whether a given number is prime or not. We are performing looping operation on it for checking a number is prime or not.
A number that is divisible only by itself and 1 is called prime number.
2, 3, 5, 7, 11, 13, 17 etc.
Scanner function is use to take the input from user in java.
import java.util.Scanner; class PrimeNumber { public static void main(String arg[]) { int n,i,p=0; Scanner sc = new Scanner(System.in); System.out.println("ENTER A NUMBER:- "); n = sc.nextInt(); for(i=2;i<=n/2;i++) { if(n%i==0) { p++; break; } } if(p==0 && n!= 1) { System.out.println(n+" IS PRIME NUMBER."); } else { System.out.println(n+" IS NOT A PRIME NUMBER."); } } }
ENTER A NUMBER:- 5 5 IS PRIME NUMBER.
Advertisment