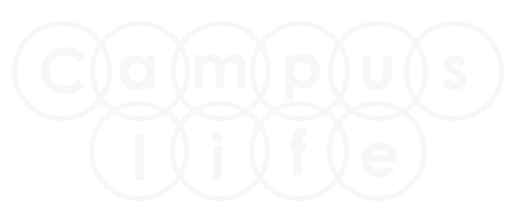
"KEEP LEARNING, DON'T STOP."
"KEEP LEARNING, DON'T STOP."
In this section you will learn reverse a numbers in java using loop. We will use three variable for reverse the numbers and simple mathematic operation we will perform to reverse the number. In this program we are taking the input from user.
Reverse mean shifting the first value to last value and shifting the last value to first value.
Let’s suppose a user will input a number ‘134’, then we will perform reverse operation on it.
Scanner function is use to take the input from user in java.
import java.util.Scanner; class Reverse { public static void main(String arg[]) { int n, temp, rev = 0; Scanner sc = new Scanner(System.in); System.out.println("ENTER A NUMBER TO REVERSE:-"); n = sc.nextInt(); temp = n; while(n != 0) { rev = rev *10; rev = rev + n%10; n = n/10; } System.out.println("AFTER REVERSE RESULT IS:- "+rev); } }
AFTER REVERSE RESULT IS:- 431
Advertisment