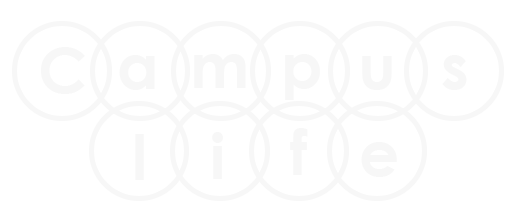
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
Array is like a Basket. We carried basket when we are going to market. In a basket we can store apple, banana, vegetables etc. Here array is same like basket.
In array we can store homogeneous (Similar type) type of data. An array is an indexed collection of homogeneous data element (value). Every value is store in array based on indexed. In array we can store multiple values.
Three types of array are in C language
In single dimensional array we can store only single data type value. Single variable but one [ ] subscript.
int a[10]; float b[10]; char c[10];
In 2D or multi-dimensional array we can store multiple data value with single variable but multiple [ ] [ ] subscript. Array brackets are called subscript.
int a[2][2] = {{5,6}, {8,3}}; //first value for row and second value for column. float b[2][2] = {{5,6}, {8,3}}; char c[2][2] = {{5,6}, {8,3}};
Very rare case people use 3 Dimensional Array. Mostly we use 1D or 2D array in C.
data_type variable_name [size_of_array/index];
int a[5]; float a[5]; char ch[20]; double d[10];
int a[]; //invalid array declaration(size manadatory) int a[-5]; //invalid (size cannot be - negative) int i=10; int a[i]; //invalid (constant expression required at declaration time)
In two ways we can initialize the Array.
Further there are two types of compile time initialization.
int a[5]; a[0]; a[1]; a[2]; a[3]; a[4];
int a[5] = {1,2,3,4,5}; //mostly we use single line initialization.
int a[] = {1,2,3,4,5}; //Valid in single line initialization int a[5] = {1,2,3}; //remaining all value become zero like 4,5 missing int a[5] = {1,2,3,4,5,6}; //invalid initialization //because array size is 5 and we are entering 6 values int a[50] = 100; //invalid but valid in c //because c doesn't have strong boundary check
In runtime array initialization we initialize the value to the array or size to the array at runtime through console window. We asking the value from user.
#include<stdio.h> #include<conio.h> void main() { int a[]5; int i; clrscr(); printf("Enter the Value of array.\n"); for(i=0;i<5;i++) { scanf("%d",&a[i]); } getch(); }
Enter the value of array. 2 3 4 5 6
We can access the element of array in two ways.
#include<stdio.h> #include<conio.h> void main() { int a[5] ={1,2,3,4,5}; int i; clrscr(); printf("Element of array:-\n"); for(i=0;i<5;i++) { printf("%d\n",a[i]); } getch(); }
Element of array:- 1 2 3 4 5
#include#include void main() { int a[5] ={1,2,3,4,5}; int i; clrscr(); printf("Element of array:-%d",a[0]); printf("\nElement of array:-%d",a[1]); printf("\nElement of array:-%d",a[2]); printf("\nElement of array:-%d",a[3]); printf("\nElement of array:-%d",a[4]); getch(); }
Element of array:- 1 Element of array:- 2 Element of array:- 3 Element of array:- 4 Element of array:- 5