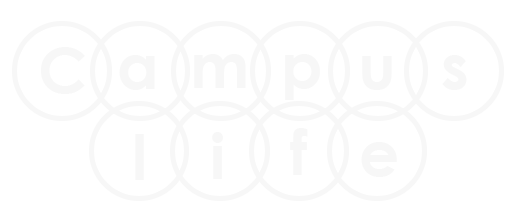
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
Union is same like as structure. Union is a one concept that used to create our own data type or you can say user define data type. Simply we use the union to create the different user define data type. Each element in a union is called member. In union memory utilization is proper.
Union size is not fixed. It's depends on number of data types in union. In union we can create multiple data types. All data value store in highest data member memory.
Union syntax is same as structure syntax. Only keyword is different.
union union_name { data_types variable_name1; data_types variable_name2; data_types variable_n; }union_variable_name;
In three ways we can create union variables in C.
union book { char bname[20]; int pages; float price; }b;
union book { char bname[20]; int pages; float price; }; union book b;
union book { char bname[20]; int pages; float price; }; void main() { union book b; //union variable declaration inside the main() function. }
When we declare the variable of union then memory allocated to union user define data type. All data store in highest memory location in union which data member have highest size. In union data store in same memory location.
#include<stdio.h> #include<conio.h> #include<string.h> union book { char bname[20]; int pages; float price; }b; void main() { clrscr(); strcpy(b.bname,"Campuslife"); b.pages=650; b.price=500.00; printf("Book Name:- %s",b.bname); printf("\nBook Pages:- %d",b.pages); printf("\nBook Price:- %f",b.price); getch(); }
Book Name:- Campuslife Book Pages:- 650 Book Price:- 500.0000
#include<stdio.h> #include<conio.h> #include<string.h> union book { char bname[20]; int pages; float price; }; union book b; void main() { clrscr(); strcpy(b.bname,"Campuslife"); b.pages=650; b.price=500.00; printf("Book Name:- %s",b.bname); printf("\nBook Pages:- %d",b.pages); printf("\nBook Price:- %f",b.price); getch(); }
Book Name:- Campuslife Book Pages:- 650 Book Price:- 500.0000
In above program we used strcpy() function to store the string or character value. Without strcpy() function you cannot store the string or character value.