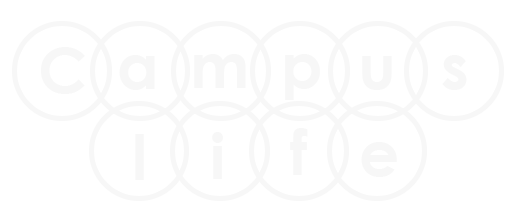
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
In C string is represented as an array of character or character array. String is a group of character enclosed within the double quotes (" ") is called as a string or string constant.
String always terminated with a null character {/0} at the end of string.
I am happy
In above example 'I am happy' is a string. When compiler compile the above string, it appends a null character /0 at the end of string.
datatype variable_name [size of array] = "any string"; char a[] = "I am happy"; or char a[10] = "I am happy"; or char a[] = {'I',' ','a','m',' ','h','a','p','p','y'}; or char a[10] = {'I',' ','a','m',' ','h','a','p','p','y'};
In above syntax of string the string is constant. Mean string is fix and you cannot take it at run time.
In string null character display as space.
scanf() function is use to read a string or any other datatype from the keyword.
The scanf() function only takes first enter word. scanf() function will automatically terminated after space or just space.
char a[10]; scanf("%s",a);
#include<stdio.h> #include<conio.h> void main() { char a[20]; clrscr(); printf("Enter your name: "); scanf("%s",a); printf("Your name is: %s",a); getch(); }
Enter yout name: Campus Life Your name is: Campus
In above example, program ignores Life string because scanf() function takes only a single string before the white space, like only Campus.
To avoid this problem C language people given function, called gets() function. This function read whole line including with white space from keyword.
#include<stdio.h> #include<conio.h> void main() { char a[20]; clrscr(); printf("Enter your name: "); gets(a); printf("Your name is: %s",a); getch(); }
Enter your name: CampusLife Your name is: CampusLife
printf() function never give you new line. So solution is.
Two function are there in C language.
gets(); //take string from keyword. puts(); //display the string on the screen or on console window.
#include<stdio.h> #include<conio.h> void main() { char a[20]; clrscr(); puts("Enter your name: "); gets(a); puts(a); getch(); }
Enter your name: Campus Life Campus Life