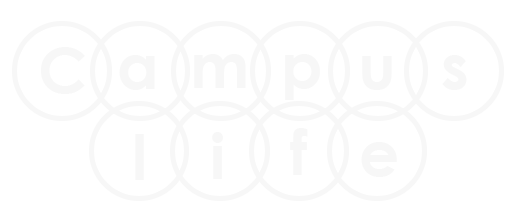
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
Control Flow Statements are also C program statement. Which are used to specify the order in which my program get executed at run time. To control the order of program we use control flow statement.
Our program execute in sequential order. But we want control the sequence of the program then we use control flow statement. We cannot write the large program using sequential programming. We only achieve it with the help of Conditional programming.
Only simple program we write in sequence order. It is not enough for large programming.
//Abstraction program void main() { int a = 10, b = 20, c; c = a + b; printf("Result is:- %d",c); getch(); }
We cannot write the large program using Sequential programming. We only achieve it with the help of conditional along with Sequential programming.
//Greater number program void main() { int a = 2, b = 5; if(a>b) { printf("A is Greater."); } else { printf("B is Greater."); } getch() }
In C we have three type of control flow statement.
conditional or branching statement | looping Statement | jumping statement |
---|---|---|
if statement | while loop statement | continue statement |
if else statement | do while loop statement | goto statement |
if else ladder statement | for loop statement | break statement |
switch case statement | return statement |
if statement also called simple if statement.
if(condition) { statement 1; }
void main() { int num; printf("Enter the any number = "); scanf("%d",&num); if(num%2==0) { pritf("Number is even."); } getch(); }
In above example you will see the even-odd number program which is without else condition.
Nested if condition mean if condition within the if condition. You can write many nested if condition it's up to you.
if(expression1) { if(expression2) { statement1; } statement2; }
If first if condition is false than it will simply come out from the all if condition. If first if condition is true than it will go to second if condition. If second if condition is also true than it will print the statement1 and statement2. If first if condition is true and second if condition is false than it will only print the statement2.
void main() { clrscr(); if(2<5) { if(4<8) { printf("Ture\n"); } printf("True"); } getch(); }
True True
void main() { clrscr(); if(2>5) { if(4>8) { printf("Ture\n"); } printf("True"); } printf("It will print nothing because both condition is false."); getch(); }
It will print nothing because both condition is false.
In if else condition executes the body of if when it is true and executes the body of else if (if) condition is false.
int num=5; if(num < 8) { printf("True"); } else { printf("False"); }
True
int num = 5; if(num > 8) { printf("True"); } else { printf("False"); }
False
Nested if else are used when there are more than one or more condition expression.
if(expression) { statement1; } else if(expression2) { statement2 } else if(expression3) { statement3 }
void main() { int a=4,b=2,c=7; clrscr(); if(a > b && a > c) { printf("a is greater."); } else if(b > a && b > c) { printf("b is greater."); } else { printf("c is greater"); } getch(); }
c is greater.