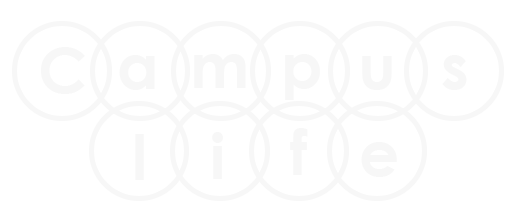
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
"HARD WORK BEATS TALENT WHEN TALENT DOSEN'T WORK HARD."
For loop is use in program when we know the number of iteration. How much iteration go long.? We exactly know the number of iteration.
for(initialization; condition; updation) { statement; }
Initialization in for loop brackets is optional. You can set the initialization outside of the loop.
initialization; for (; condition; updation) { statement }
Condition is also optional in for loop brackets. You can set the condition outside of the loop
initialization; condition; for (; ; updation) { statement; }
Updation is also optional in for loop brackets.
But semi-column ( ; ) is necessary in for loop bracket.
initialization; condition; for (; ;) { statement; updation; }
for loop only one time go inside the initialization.
for loop check condition until it not become false.
Execute the body portion.
Next it will go for updation.
step 2, step 3 and step 4 go until condition not become false. It will keep performing the iteration until condition not become false. At last it will come out from the loop body.
#include<stdio.h> #include<conio.h> void main() { int i; printf("Printing the number from 1 to 10.\n"); for(i=1; i<=10; i++) { printf(" %d \n",i); } getch(); }
Printing the number from 1 to 10. 1 2 3 4 5 6 7 8 9 10
#include<stdio.h> #include<conio.h> //program of table 2. void main() { int i; int fact; clrscr(); for(i=1; i<=10; i++) { fact = i*2; printf("%d\n",fact); } getch(); }
2 4 6 8 10 12 14 16 18 20